Post-order Traversal: Left, Right, Root!
- Explain post-order traversal.
This is the strategy for post-order traversal:
For every node, visit its left subtree, then visit its right subtree, then visit the node.
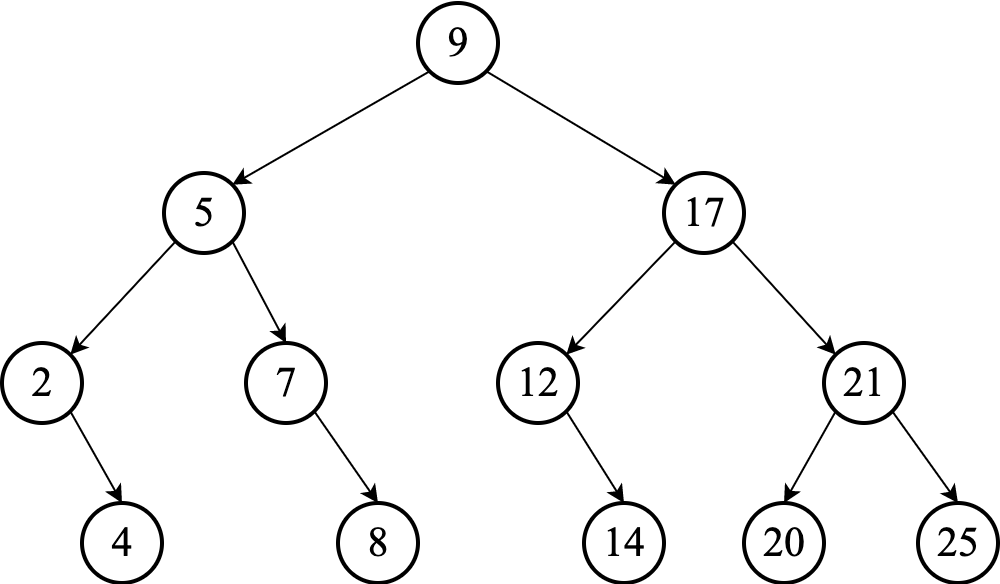
Following the strategy above will generate this sequence:
Explanation
We start with the root, , but we must first visit its left subtree. So we move to , but we must first visit its left subtree. So we move to . We must visit the left subtree of , but there is none. So we move to visit the right subtree of , which is rooted at . We must, however, first visit the left subtree of . Since there is no left subtree to , we move to visit its right subtree. There is, however, no subtree to the right of . So we can visit itself. Now we are done with the right subtree of , so we can visit itself.
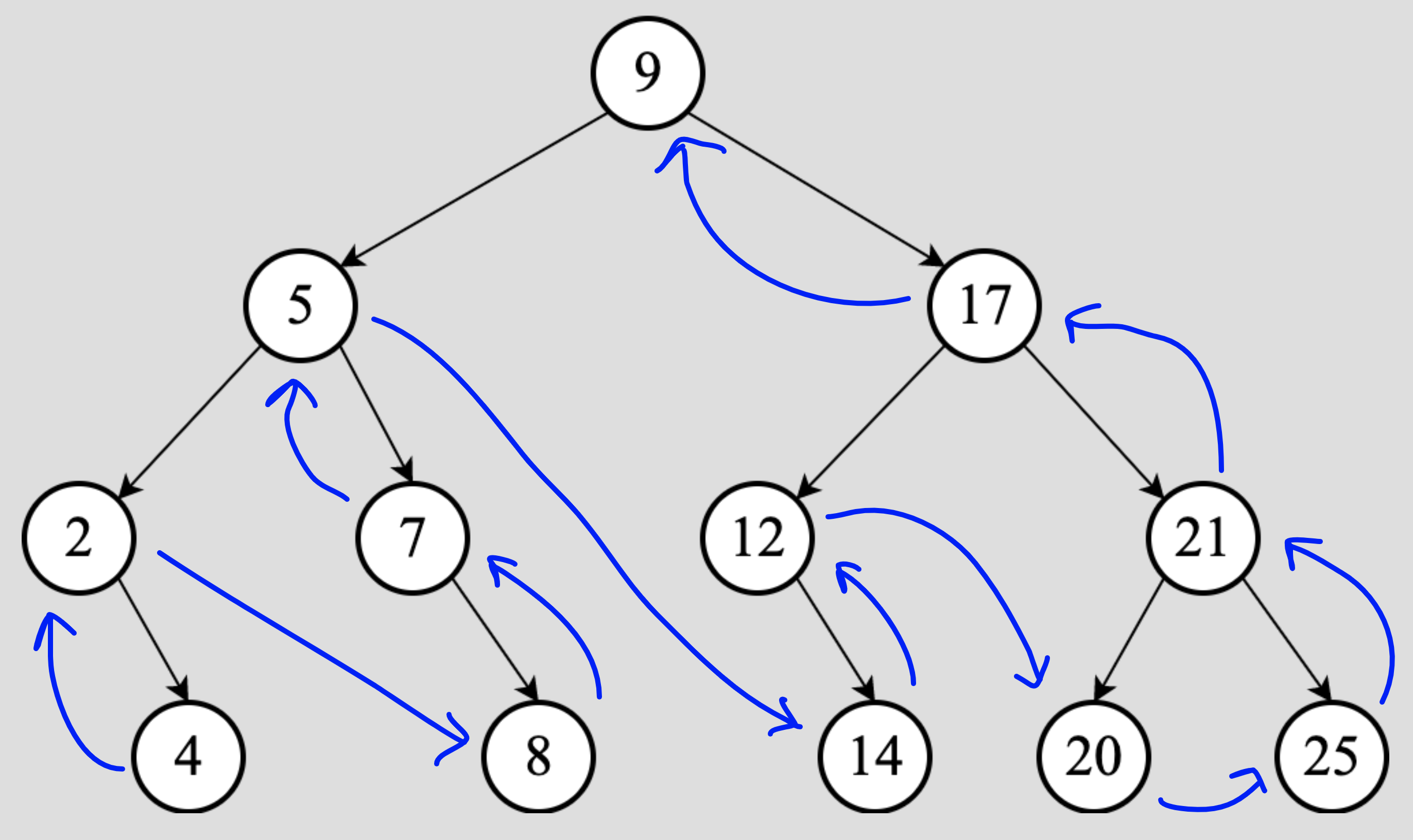
Now we are done with the left subtree of . We must visit the right subtree of now. So we move to , but we must first visit its left subtree. There is no subtree to the left of , though. So we can move to the right subtree of . Therefore, we move to . We must now visit the left subtree of , but there is none. So we move to visit the right subtree of , but there is none. We can, therefore, visit itself. Once is visited, we are done with the right subtree of , so we can visit itself. Once we have visited , we are done with the right subtree of , so we can visit itself. Once we have visited , we are done with the left subtree of , so we must move to visit the right subtree of
Here is a recursive definition of post-order traversal: for each node, recursively perform post-order traversal of its children (the subtrees rooted at its left and right child, in that order), then visit the node itself.
Resources
- Post-order tree traversal in 2 minutes on YouTube.